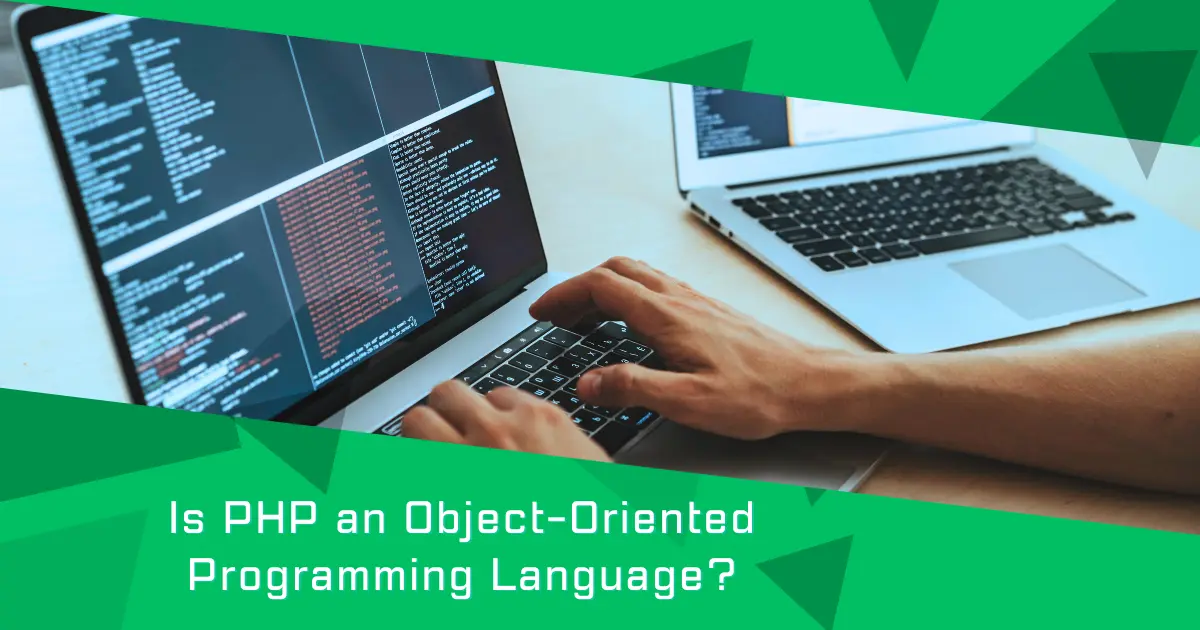
Is PHP an Object-Oriented Programming Language?
Created in the mid-1990s, PHP-short for Personal Home Page Tools-is a server-side scripting programming language that has grown both organically and purposefully over time. Despite its lineage as a procedural language, PHP provides support for many object-oriented programming (OOP) features. These days PHP is known to be a compelling Object Oriented Language and it gives us the scope of choosing from all its OOP features that are being used by developers while they write large and maintainable as well as scalable applications. However, why is PHP considered to be an object-oriented language and how does it stand compared to other OOP languages? In this article, we will explore the ground of PHP and OOP to answer those questions.
History of PHP and OOP
PHP becoming an OOP language In my own words…. The original PHP versions were designed as procedural languages with simple scripting possibilities for web development tasks. As the language evolved, its capabilities advanced as well. So PHP 4 was introduced having basic OOP features in it letting developers define the classes and objects. PHP 5 took that all-important monumental leap, in the introduction of a complete OOP-set which added more class-hierarchy levels for inheritance with refined support, and an improved object model. Later versions further optimized these features (such as PHP 7 and now with PHP 8), eventually making OOP a fundamental aspect of the language.
What is Object-Oriented Programming?
Object-oriented programming is a way to define types of data as objects instead of functions and logics. It follows that in OOP, objects are instances of classes which is the blueprint for creating an object. This is because the paradigm favors reusability, modularity and extensibility-important attributes for any large codebase of software.
How OOP evolved in PHP
- Procedural Roots: PHP 3 and Prior
- One characteristic of the early PHP versions was its relatively small focus on object-oriented programming opposed to procedural scripting.
- Basic OOP Features – Introduction to PHP 4
- The most important feature in PHP 4 is, of course object -oriented programming and its basic class & objects support.
- Significant Improvements in OOP Support (PHP 5)
- Full OOP with encapsulation (private, protected and public accessors), magic methods etc.
- Improvements in OOP Features, PHP 7 and Up
- While PHP 7 and now with changes in PHP8 it added type declarations, some magic methods to enhance the experience of OOP.
Core OOP Concepts in PHP
PHP (at least version 5 and above) support OOP, however it lacks at few core concepts of any object-oriented programing language;
- Classes and Objects: The basics of OOP in PHP
- Inheritance: One class has the ability to inherit properties from another.
- Encapsulation – defined as the mechanism that restrict certain components, exposing a well planned-controlled interface.
- Polymorphism: It means that objects of child class can be handled by the parent domain.
- Abstraction : This allows to create abstract classes and interfaces which cannot be instantiated.
PHP OOP Syntax and Features
- Creating Objects in PHP Classes: A class is defined using the keyword class, and objects are created by applying a new statement.
- Methods and Properties: A method is a function defined within a class, and property are variables.
- Constructors and Destructors: These are special methods called automatically when an object is created or deleted.
- Access Modifiers: These are the keywords like Public, Private and Protected which determine visibility of class properties & methods.
- Static Properties and Methods: Static properties belong to the class itself, rather than any object instance.
Advanced OOP Features in PHP
PHP Advanced OOP features:
- Interfaces – you define methods that a class must implement
- Traits: Offer how we can reuse code for languages that do not support multiple inheritance such as PHP.
- NAMESPACES: Keep the naming conflict at bay by putting code into namespaces.
- Abstract Classes: Abstract classes are base classes that cannot be instantiated.
- Magic Methods: Special methods such as __construct, __destruct or even __get provide extended functionality.
Examples of OOP in PHP
- Simple Class Example
- code
class Car
{ public $color;
public $model;
public function __construct($color, $model)
{ $this->color = $color; $this->model = $model;
} public function message()
{ return "My car is a " . $this->color . " " . $this->model . ".";
} } $myCar = new Car("red", "Toyota");
echo $myCar->message();
- Inheritance and Method Overriding:
- code
class Animal
{ public function makeSound() { echo "Animal sound";
}
} class Dog extends Animal { public function makeSound()
{ echo "Bark"; }
} $myDog = new Dog();
$myDog->makeSound();
// Outputs: Bark
- Using Interfaces and Traits
- code
interface Logger
{ public function log($message);
} class FileLogger implements Logger
{ public function log($message) { echo "Logging to a file: " . $message; }
} $logger = new FileLogger();
$logger->log("Hello, world!");
Benefits of Using OOP in PHP
- Reusability: One of the most important features provided by OOP is creating reusable code components.
- Better Maintainability: Code segments are organized in terms of classes, so it is quick and convenient to keep them updated.
- Data Security: Encapsulation helps to secure data from unauthorized access.
- Facilities debugging and Testing – the object-oriented approach is responsible for unit testing.
Top Myths About PHP And Object Oriented Programming
- PHP is not just OOP: PHP began as an unproved language, but now PED is open to international application.
- OOP is complex for a beginners: Well, yes OOP concepts are not so easy to understand but it has great benefits too once you get accustomed with them.
- When to Use Procedural PHP vs. OOP PHPOOP: is generally better for complex, scalable apps
Comparing PHP OOP with Other Object Oriented Programming (OOP) Languages
- PHP vs. Java: Java is a proper OOP language while PHP is multi-paradigm, it can function as procedural programming or Object-oriented.
- Python and PHP support OOP: Python, but the simplicity of word design is much better.
- PHP vs. C++: While C++ provides a better ability to fine-tune at the low level, it is typically used with web development and PHP.
C++ Programming for Embedded Systems…
Applications of OOP in Real World Using PHP
- Examples WordPress (Content Management System): A popular CMS with a lot of OOP principles.
- E-commerce Platforms: One of the leading e-Commerce platform, Magento is developed with OOP structure in PHP.
- Web Frameworks: OOP makes it easier to develop powerful web frameworks like Laravel and Symfony.
Problem, probleme – Object-oriented programming with PHP
- Performance: sometimes OOP can come with some performance overhead but in general, it is compensated by the advantages.
- Learning Curve: Some developers may find it difficult to understand OOP concepts early on, giving rise the Learning curve.
- Compatibility and Legacy Code: Migrating procedural code from a legacy to OOP can be an arduous process but it may yield dividends on the long run.
Best Practices for OOP in PHP
- Some common design patternsDesign Patterns: Singleton, Factory and Observer pattern
- Follow the SOLID Principles: Write code based on the principles of Object-Oriented Design, aiming for flexiblity and maintainability.
- MVC application From here: Insert this as needed in places where documentation is available Step 1 In IcustomerRepository.
PHP has come a long way from its procedural beginnings to become a robust object-oriented language. The introduction and enhancement of OOP features have enabled PHP developers to create more complex, scalable, and maintainable applications. Whether you’re building a small personal project or a large-scale enterprise application, PHP’s OOP capabilities provide the tools you need to succeed. As the language continues to evolve, it’s clear that OOP will remain a central aspect of PHP development.